Table of Contents
I've built a few things on .net core over the last while. I like many people are excited by what it can offer us. This post is a quick summary of the things I've found or that have caused me an issue. I will be updating it as I find new stuff.
.Net Core
As of writing this post .net core 3.1 is now the long term supported standard. Which basically means it's the one that will be supported for 3 years.
This doesn't mean that your applications or websites built in .net core 1.1 or 2.0 or which ever you picked will stop working. Far from it as is usually the case with using Mircosofts tools they will work until the rest of us are no more and the coffee beans have risen up and taken over.
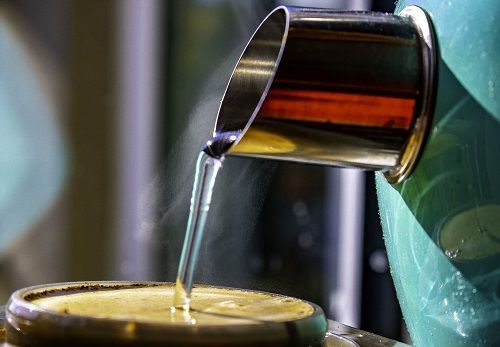
So do not panic you don't have to actually do this. But if you want to develop the application further it would be a good idea to do the upgrade.
.Net Core 3.1
I have been upgrading a few of my own applications to .Net core 3.1 and mostly it has been pretty simple and straightforward but I have a few gotcha moments that something didn't work right.
AllowSynchronousIO
I have a few API methods that read the body of the request.
Stream req = Request.Body;
string json = new StreamReader(req).ReadToEnd();
var content = JsonConvert.DeserializeObject<dynamic>(json);
We all know the code it reads the request stream and then converts the JSON in the body into an object so I can process it further.
In .Net Core 3.1 this doesn't work because by default the AllowSynchronousIO is disabled.
To fix this in the Startup.cs in the "ConfigureServices"
public void ConfigureServices(IServiceCollection services)
{
services.Configure<IISServerOptions>(options =>
{
options.AllowSynchronousIO = true;
});
services.Configure<KestrelServerOptions>(options =>
{
options.AllowSynchronousIO = true;
});
services.AddControllersWithViews();
}
I set options on both IISServer and on Kestrel, I run alot of my .net core applications in docker containers so these use Kestrel, while debugging usually uses IIS. But it's really cool I can set different options for different engines.
All we're doing here is enabling AllowSynchronousIO. This allows our code to read the body.
Note: I haven't figured out anything else that this might cause issues with but I think there might be a few. I'll update this post if I come across more or let me know if you found something.
Globalization Invariant Mode - Docker
This one has turned out to be a really weird issue, but it only happened for me when I launched the docker application. Debugging from VS it all ran fine but as soon as I built the docker container and deployed I was getting this error any time I tried to get to the database.
I still don't have a full understanding of what is behind this. The fix I found was moving off from the alpine Docker images and over to bionic and now I don't have the issue anymore.
I'm still digging into actually why it is happening. I've only found it when I switched to the Alpine 3.1 images. It doesn't happen to the 3.0 images.
FROM mcr.microsoft.com/dotnet/core/sdk:3.1-bionic AS build
That's it for now. I'll be updating this post as I find any new things. The upgrade process has been really simple and straight forward so far. But I'm sure there are a few other things that might trip me up. Let me know if you have found anything that I should add.